Learn how to handle JSON in PHP with json_encode() and json_decode(). Convert arrays to JSON, parse JSON data, and manipulate it efficiently.
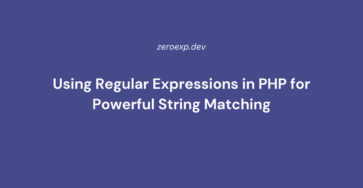
Using Regular Expressions in PHP for Powerful String Matching
Learn how to use regular expressions in PHP for powerful string matching and pattern recognition. Master preg_match(), preg_replace(), and preg_split() for efficient text processing.
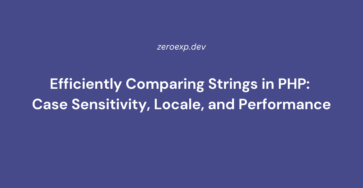
Efficiently Comparing Strings in PHP: Case Sensitivity, Locale, and Performance
Learn how to compare strings in PHP efficiently, including case-sensitive and case-insensitive comparisons, locale-aware comparisons, and performance considerations.
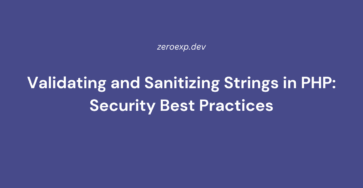
Validating and Sanitizing Strings in PHP: Security Best Practices
Learn how to validate and sanitize strings in PHP to prevent security vulnerabilities like SQL injection and XSS. Use built-in PHP functions for safe input handling.
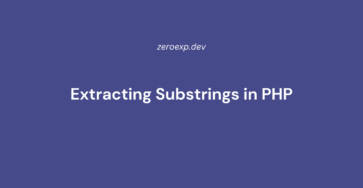
Extracting Substrings in PHP
Excerpt (Meta Description)
Learn how to extract substrings in PHP using substr(), mb_substr(), and alternative methods. Handle UTF-8 characters correctly and manipulate strings efficiently.
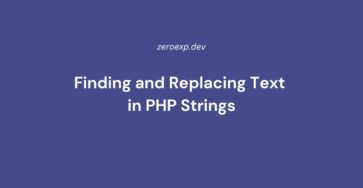
Finding and Replacing Text in PHP Strings: str_replace() vs. preg_replace()
Learn how to find and replace text in PHP using str_replace() and preg_replace(). Understand their differences and use cases for efficient text processing.
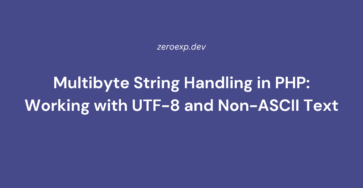
Multibyte String Handling in PHP: Working with UTF-8 and Non-ASCII Text
Learn how to handle multibyte strings in PHP using UTF-8. Work with non-ASCII characters safely using mbstring functions like mb_strlen(), mb_substr(), and more.
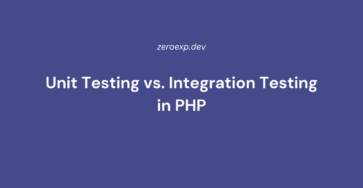
Unit Testing vs. Integration Testing in PHP: Key Differences
Understand the key differences between unit testing and integration testing in PHP. Learn when to use each type for better software quality and reliability.
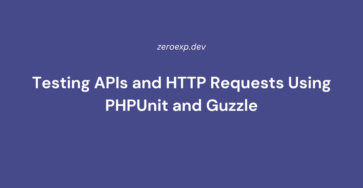
Testing APIs and HTTP Requests Using PHPUnit and Guzzle
Learn how to test APIs and HTTP requests using PHPUnit and Guzzle. Verify API responses, handle errors, and mock HTTP requests for reliable testing.
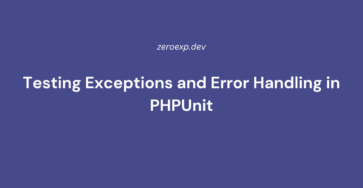
Testing Exceptions and Error Handling in PHPUnit
Learn how to test exceptions and error handling in PHPUnit. Verify that your PHP code correctly throws and handles exceptions using PHPUnit’s built-in methods.
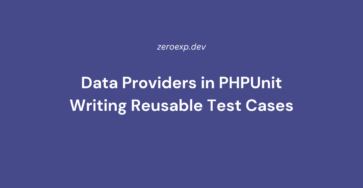
Data Providers in PHPUnit: Writing Reusable Test Cases
Learn how to use data providers in PHPUnit to write efficient and reusable test cases. Improve test coverage by running multiple scenarios dynamically.
Testing Private and Protected Methods in PHPUnit
Learn how to test private and protected methods in PHPUnit. Use reflection and proper testing strategies to ensure private logic works as expected.